Launchファイル¶
ROSでは複数のノードを同時に立ち上げて、それぞれのノードからデータを送受信したりしています。
今まではros2 run
コマンドで1つ1つノードを立ち上げていましたが、実際には複数のノードをLaunchファイルというもので起動します。
このLaunchファイルからトピック名やノード名、パラメータの値を変更出来るので非常に便利です。
注釈
ここでは
トピック通信
で作ったhello_topic
パッケージのiteration_node
ノードと
パラメータ
で作ったhello_param
パッケージのmultiply_node
ノードを使います。
まだ作ってない人は先に、そちらをご覧ください。
今回の目標¶
今回は以下のようなLaunchファイルをもつhello_launch
パッケージを作りましょう。
hello.launch.py
以下の2つのノードを立ち上げるLaunchファイル
hello_topic
パッケージのiteration_node.py
hello_topic
パッケージのdouble_node.py
two_double.launch.py
以下の3つのノードを立ち上げるLaunchファイル
hello_topic
パッケージのiteration_node.py
hello_topic
パッケージのdouble_node.py
hello_topic
パッケージのdouble_node.py
double_node
ノードをquad_node
ノードに変更/number
トピックを/double_number
トピックにリマップ/double_number
トピックを/quad_number
トピックにリマップ
double_and_multiply.launch.py
以下の3つのノードを立ち上げるLaunchファイル
hello_topic
パッケージのiteration_node.py
hello_topic
パッケージのdouble_node.py
hello_param
パッケージのmultiply_node.py
/number
トピックを/double_number
トピックにリマップm_number
パラメータを4
に変更
Tip
今回はPythonでLaunchファイルを書きますが、YAMLやROS 1時代のXMLでもLaunchファイルを書けます。 詳しくは公式のチュートリアル(英語)を読んでください。
パッケージの作成¶
ros2 pkg create --build-type ament_cmake hello_launch
Launchファイルを保存するlaunch
ディレクトリを作成します。
cd hello_launch
mkdir launch
CMakelists.txtの編集¶
以下の3行をCMakelists.txt
に追加
# 追加
install(
DIRECTORY launch
DESTINATION share/${PROJECT_NAME}
)
最終的なCMakelists.txt
は以下のようになります。
cmake_minimum_required(VERSION 3.8)
project(hello_launch)
if(CMAKE_COMPILER_IS_GNUCXX OR CMAKE_CXX_COMPILER_ID MATCHES "Clang")
add_compile_options(-Wall -Wextra -Wpedantic)
endif()
# find dependencies
find_package(ament_cmake REQUIRED)
# uncomment the following section in order to fill in
# further dependencies manually.
# find_package(<dependency> REQUIRED)
# 追加
install(
DIRECTORY launch
DESTINATION share/${PROJECT_NAME}
)
if(BUILD_TESTING)
find_package(ament_lint_auto REQUIRED)
# the following line skips the linter which checks for copyrights
# comment the line when a copyright and license is added to all source files
set(ament_cmake_copyright_FOUND TRUE)
# the following line skips cpplint (only works in a git repo)
# comment the line when this package is in a git repo and when
# a copyright and license is added to all source files
set(ament_cmake_cpplint_FOUND TRUE)
ament_lint_auto_find_test_dependencies()
endif()
ament_package()
hello.launch.pyのコード¶
launch/hello.launch.py
を以下の内容で書き込む。
from launch import LaunchDescription
from launch_ros.actions import Node
def generate_launch_description():
return LaunchDescription([
Node(
package='hello_topic',
executable='iteration_node',
name='iteration_node',
),
Node(
package='hello_topic',
executable='double_node',
name='double_node',
),
])
two_double.launch.pyのコード¶
launch/two_double.launch.py
を以下の内容で書き込む。
from launch import LaunchDescription
from launch_ros.actions import Node
def generate_launch_description():
return LaunchDescription([
Node(
package='hello_topic',
executable='iteration_node',
name='iteration_node',
),
Node(
package='hello_topic',
executable='double_node',
name='double_node',
),
Node(
package='hello_topic',
executable='double_node',
name='quad_node',
remappings=[
('/number', '/double_number'),
('/double_number', '/quad_number'),
],
),
])
double_and_multiply.launch.pyのコード¶
launch/double_and_multiply.launch.py
を以下の内容で書き込む。
from launch import LaunchDescription
from launch_ros.actions import Node
def generate_launch_description():
return LaunchDescription([
Node(
package='hello_topic',
executable='iteration_node',
name='iteration_node',
),
Node(
package='hello_topic',
executable='double_node',
name='double_node',
),
Node(
package='hello_param',
executable='multiply_node',
name='multiply_node',
remappings=[
('/number', '/double_number'),
],
parameters=[
{'m_number': 4},
]
),
])
ビルドと実行¶
cd ~/my_ws
colcon build
source install/setup.bash
ros2 launch
コマンドでLaunchファイルを立ち上げましょう。
立ち上がったらrqt_graph
でノード間の通信の様子やrqt
を使ってトピックを見てみましょう。
Tip
rqt
でトピックを見るにはPlugins
タブからTopics
、Topics
からTopic Monitor
を選択してトピックの様子を見てみましょう。
hello.launch.py¶
ros2 launch hello_launch hello.launch.py
ノード間の通信の図は既に Hello world で紹介したグラフと同じなので省略します。
two_double.launch.py¶
ros2 launch hello_launch two_double.launch.py
rqt_graph
rqt

rqt_graph¶
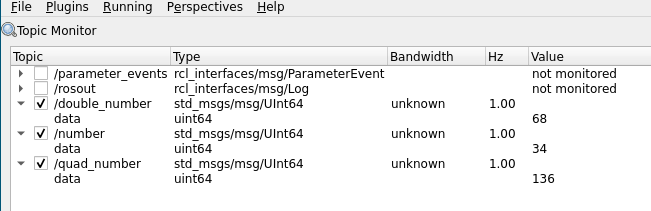
rqtでトピックを見た様子¶
/number
トピックの値を2倍したものが/double_number
へ、/double_number
トピックの値をさらに2倍したものが/quad_number
へ送られているのが分かります。
double_and_multiply.launch.py¶
ros2 launch hello_launch double_and_multiply.launch.py
rqt_graph
rqt

rqt_graph¶
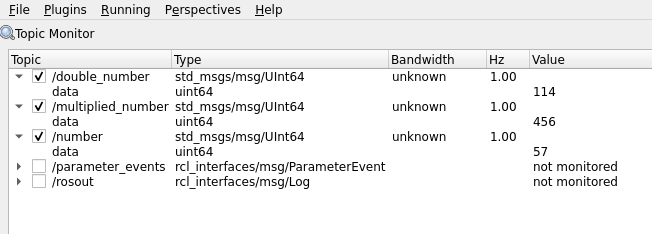
rqtでトピックを見た様子¶
/number
トピックの値を2倍したものが/double_number
へ、/double_number
トピックの値をさらにパラメータで指定した4倍にしたものが/multiplied_number
へ送られているのが分かります。